I improved the rackspace/script to be working with a full 9-set of drawbars and faders while hiding the “uncontrolled” group of widgets…

Description:
A Rackspce Script is used to have a single set of hardware controllers (say, faders) move two diffrent groups of widgets, dependent on the position of a “destination switch” widget.
The script will send the incoming CC# messages to either the group of drawbars or the group of faders, while at the same time, the widgets that are not to be controlled will be hidden, so you will only see (and control) one single type of widgets at a time (drawbars or faders).
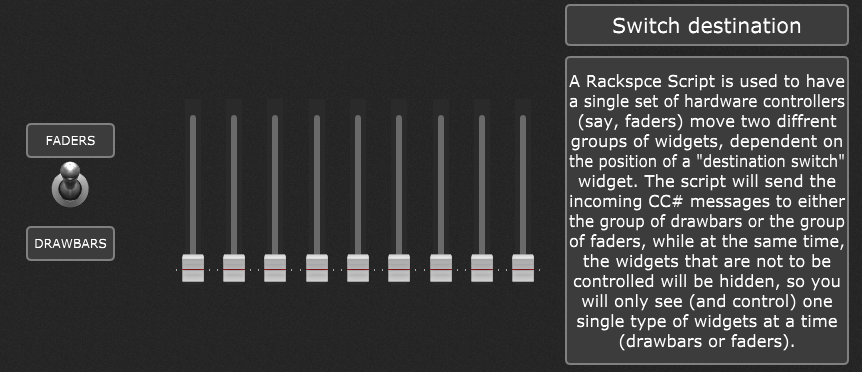
switch destination drawbars-faders.gig (292.6 KB)
The script code for the improved version:
var
MIDI_in : MidiInBlock
swt_changeDest : widget //switch destination of CC#
fdr1, fdr2, fdr3, fdr4, fdr5, fdr6, fdr7, fdr8, fdr9 : widget // faders
drwb1, drwb2,drwb3, drwb4, drwb5, drwb6, drwb7, drwb8, drwb9 : widget // drawbars
ccNrs : integer array = [20, 21, 22, 23, 24, 25, 26, 27, 28] // incoming cc# for the nine faders/drawbars
faders_arr : widget array = [fdr1, fdr2, fdr3, fdr4, fdr5, fdr6, fdr7, fdr8, fdr9] // array for white faders
drawbars_arr : widget array = [drwb1, drwb2,drwb3, drwb4, drwb5, drwb6, drwb7, drwb8, drwb9] // array for black faders
// User function to move either the black or white faders, depending on the destination-switch position
function MoveFader (index : integer, ccNr : integer, ccVal : integer)
If GetWidgetValue(swt_changeDest) >0.6 Then
SetWidgetValue (faders_arr[index], MidiToParam(ccVal))
Else
SetWidgetValue (drawbars_arr[index], MidiToParam(ccVal))
End
SendNow (MIDI_in, MakeControlChangeMessage(ccNr, ccVal))
End
// user function to hide/unhide a group of faders
function HideThem (groupToHide : widget array, groupToShow : widget array)
var index : integer
For index = 0; index< Size(groupToHide); index = index +1 Do
SetWidgetHideOnPresentation(groupToHide[index], true)
SetWidgetHideOnPresentation(groupToShow[index], false)
end
End
//Called when a CC message is received at some MidiIn block
On ControlChangeEvent(ccMsg : ControlChangeMessage) from MIDI_in
var
ccNr : integer = GetCCNumber(ccMsg)
ccVal : integer = GetCCValue(ccMsg)
Select
ccNr == ccNrs[0] do MoveFader (0, ccNr, ccVal) //CC# array item 1
ccNr == ccNrs[1] do MoveFader (1, ccNr, ccVal) //CC# array item 2
ccNr == ccNrs[2] do MoveFader (2, ccNr, ccVal) //CC# array item 3
ccNr == ccNrs[3] do MoveFader (3, ccNr, ccVal) //CC# array item 4
ccNr == ccNrs[4] do MoveFader (4, ccNr, ccVal) //CC# array item 5
ccNr == ccNrs[5] do MoveFader (5, ccNr, ccVal) //CC# array item 6
ccNr == ccNrs[6] do MoveFader (6, ccNr, ccVal) //CC# array item 7
ccNr == ccNrs[7] do MoveFader (7, ccNr, ccVal) //CC# array item 8
ccNr == ccNrs[8] do MoveFader (8, ccNr, ccVal) //CC# array item 9
// as many as you need
//Optionally include this for when none of the above matched
True do SendNow (MIDI_in, MakeControlChangeMessage(ccNr, ccVal))
End
End
// use "destination switch" to trigger the hide/unhide user function
On WidgetValueChanged (swtVal : double) from swt_changeDest
If swtVal>0.6 then
HideThem (drawbars_arr, faders_arr) // hide drawbars, show faders
Else
HideThem (faders_arr, drawbars_arr) // hide faders, show drawbars
End
End